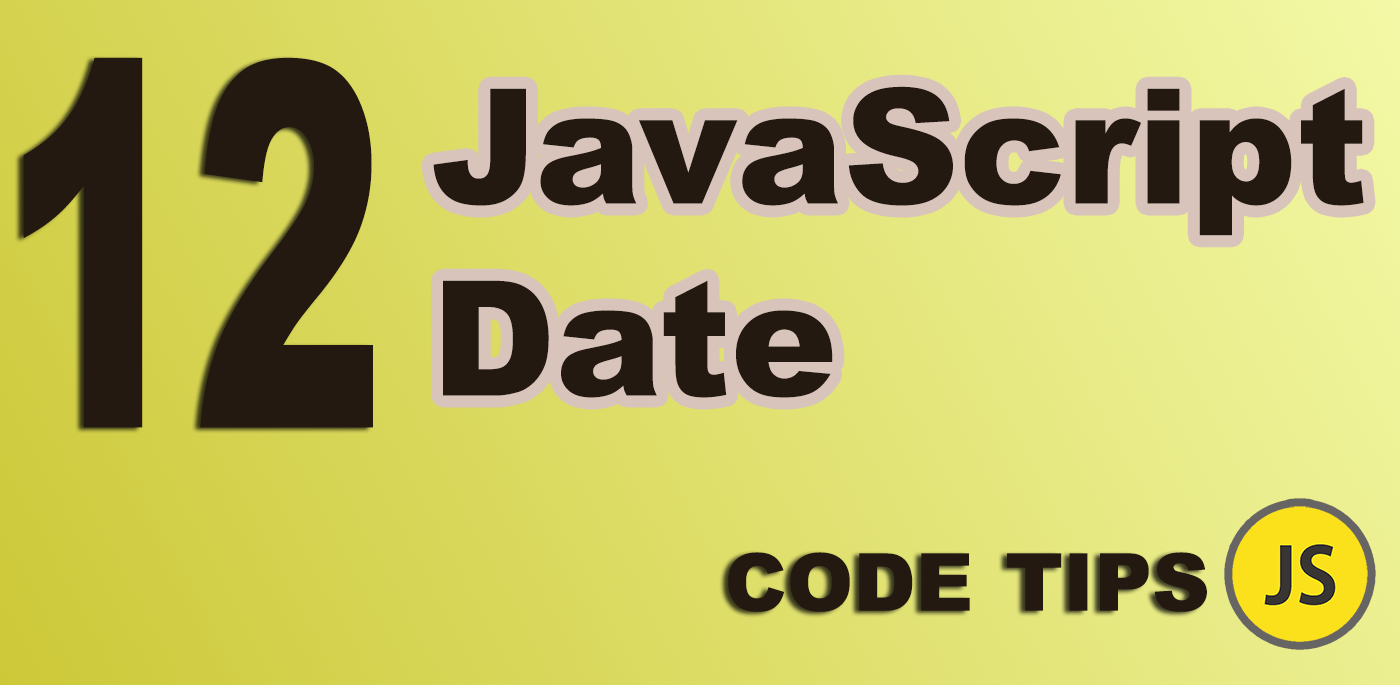
Calculate the number of difference days between two dates
const diffDays = (date, otherDate) => Math.ceil(Math.abs(date - otherDate) / (1000 * 60 * 60 * 24));
//Example
diffDays(new Date('2020-12-19'), new Date('2022-01-01')); //378
Compare two dates
// `a` and `b` are `Date` instances
const compare = (a, b) => a.getTime() > b.getTime();
//Example
compare(new Date('2022-03-30'), new Date('2022-01-01')); //true
Determine one year from now
const addOneYear = ((d) => new Date(d.setFullYear(d.getFullYear() + 1)))(new Date());
//Example
new Date(addOneYear); //Sun Dec 17 2023 19:29:43 GMT+0530 (India Standard Time)
Convert a date to YYYY-MM-DD format
// `date` is a `Date` object
const formatYmd = (date) => date.toISOString().slice(0, 10);
//Example
formatYmd(new Date()); // 2022-12-17
Get the tomorrow date
const tomorrow = new Date(new Date().valueOf() + 1000 * 60 * 60 * 24);
//Example
new Date(tomorrow); //Sun Dec 18 2022 19:26:57 GMT+0530 (India Standard Time)
Get the yesterday date
const yesterday = new Date(new Date().valueOf() - 1000 * 60 * 60 * 24);
//Example
new Date(yesterday); //Fri Dec 16 2022 19:28:55 GMT+0530 (India Standard Time)
Get the month name of a date
// `date` is a Date object
const getMonthName = (date) => ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', ' November', 'December'][date.getMonth()];
//Example
Date(getMonthName); //'Sat Dec 17 2022 19:33:58 GMT+0530 (India Standard Time)'
Get the weekday of a date
// `date` is a Date object
const getWeekday = (date) => ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'][date.getDay()];
//Example
Date(getWeekday); //'Sat Dec 17 2022 19:35:36 GMT+0530 (India Standard Time)'
Calculate the number of months between two dates
const monthDiff = (startDate, endDate) => Math.max(0, (endDate.getFullYear() - startDate.getFullYear()) * 12 - startDate.getMonth() + endDate.getMonth());
//Example
monthDiff(new Date('2022-01-01'), new Date('2023-01-01')); //12
Get the total number of days in a year
const numberOfDays = (year) => (new Date(year, 1, 29).getDate() === 29 ? 366 : 365);
//Example
numberOfDays(); //365
Get the current quarter of a date
const getQuarter = (d = new Date()) => Math.ceil((d.getMonth() + 1) / 3);
//Example
getQuarter() //4
Get the last date in the month of a date
const getLastDate = (d = new Date()) => new Date(d.getFullYear(), d.getMonth() + 1, 0);
//Example
getLastDate(); // Sat Dec 31 2022 00:00:00 GMT+0530 (India Standard Time)
Write a comment ...