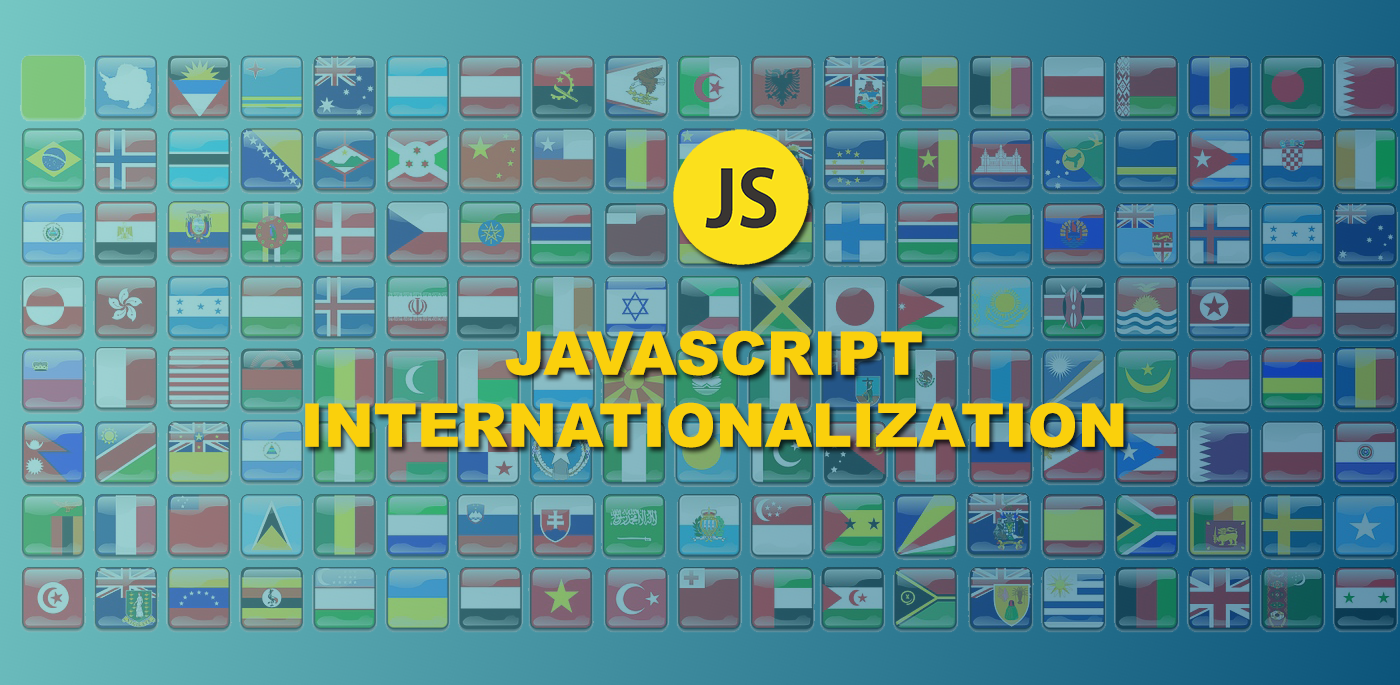
Internationalization (i18n) refers to the process of designing and developing a software application or website in such a way that it can be easily adapted to different languages and regions. This involves a number of tasks, including:
Separating language-specific content and behavior from the rest of the codebase.
Providing a way to switch between different languages and regions at runtime.
Supporting right-to-left and left-to-right text direction.
Handling differences in date, time, and number formatting conventions between regions.
Providing translations for all language-specific content.
There are a number of libraries and tools that can help with internationalization in JavaScript, such as the Intl
object and the i18next
library.
The Intl
object is a built-in object in JavaScript that provides internationalization support, including support for formatting numbers, dates, and currencies according to the conventions of a specific locale.
The i18next
library is a popular third-party library that provides a comprehensive set of tools for internationalization, including support for pluralization, interpolation, and handling of language-specific content. It also provides an easy-to-use API for switching between languages and regions at runtime.
Intl.getCanonicalLocales() Method
The Intl.getCanonicalLocales()
method is a method of the Intl
object in JavaScript that takes an array of locale strings as input and returns an array of their canonicalized form.
The canonical form of a locale string is a standardized representation of the locale that is used internally by the Intl
object. It is based on the Unicode Locale Identifier (ULI) format, which is a standardized way of representing the language, region, and other locale-specific details of a locale.
Here’s an example of how you might use the Intl.getCanonicalLocales()
method:
const locales = ['en-US', 'fr-FR', 'de-DE'];
const canonicalLocales = Intl.getCanonicalLocales(locales);
// canonicalLocales will be ['en-US', 'fr-FR', 'de-DE']
You can also pass a single string as the input to the Intl.getCanonicalLocales()
method:
const locale = 'en-US';
const canonicalLocale = Intl.getCanonicalLocales(locale);
// canonicalLocale will be 'en-US'
The Intl.getCanonicalLocales()
method is useful for ensuring that the locales you are using are in a standardized form that is recognized by the Intl
object. It can also be used to filter out any invalid or unsupported locales that may be present in the input array.
Intl.supportedValuesOf() Method
The Intl.supportedValuesOf()
method is a method of the Intl
object in JavaScript that takes a property name and an optional options object as input and returns an array of the values that are supported for that property by the Intl
object.
For example, you can use the Intl.supportedValuesOf()
method to get an array of the values that are supported for the localeMatcher
property, which determines how the Intl
object should match the requested locale to the available locales:
const supportedLocaleMatchers = Intl.supportedValuesOf('localeMatcher');
// supportedLocaleMatchers will be ['lookup', 'best fit']
You can also use the Intl.supportedValuesOf()
method to get an array of the values that are supported for the calendar
property, which determines the calendar system to be used for formatting and parsing dates and times:
const supportedCalendars = Intl.supportedValuesOf('calendar');
// supportedCalendars will be ['buddhist', 'chinese', 'coptic', 'ethiopia', 'ethiopic', 'gregory', 'hebrew', 'indian', 'islamic', 'islamic-civil', 'islamic-rgsa', 'islamic-tbla', 'islamic-umalqura', 'japanese', 'persian', 'roc'
You can pass an options object as the second argument to the Intl.supportedValuesOf()
method to specify the values that you are interested in. For example, you can use the locale
property of the options object to specify the locale to use for the lookup:
const options = {locale: 'en-US'};
const supportedTimeZones = Intl.supportedValuesOf('timeZone', options);
// supportedTimeZones will be an array of the time zones that are supported for the 'en-US' locale
The Intl.supportedValuesOf()
method is useful for checking which values are supported by the Intl
object for a given property, and for selecting the appropriate values to use when creating an Intl.DateTimeFormat
or Intl.NumberFormat
object.
Intl.Collator
The Intl.Collator
object is a part of the Intl
object in JavaScript that provides support for comparing strings according to the conventions of a specific locale. It allows you to create a collator object that can be used to compare strings in a case-sensitive or case-insensitive manner, and to specify the collation strength and sensitivity.
Here’s an example of how you might use the Intl.Collator
object to create a collator object that compares strings in a case-insensitive manner:
const collator = new Intl.Collator(['en-US'], {sensitivity: 'accent'});
const strings = ['résumé', 'Resume', 'resume'];
strings.sort(collator.compare);
// strings will be ['resume', 'Resume', 'résumé']
In this example, the Intl.Collator
object is used to create a collator object for the en-US
locale, with sensitivity set to accent
. The compare
method of the collator object is then used to sort an array of strings in a case-insensitive manner, taking into account accent differences.
You can also use the Intl.Collator
object to create a collator object that compares strings in a case-sensitive manner:
const collator = new Intl.Collator(['en-US'], {sensitivity: 'case'});
const strings = ['résumé', 'Resume', 'resume'];
strings.sort(collator.compare);
// strings will be ['Resume', 'resume', 'résumé']
In this example, the sensitivity is set to case
, so the comparison is case-sensitive.
You can also use the Intl.Collator
object to specify the collation strength and sensitivity when comparing strings. For example, you can use the strength
property to specify the collation strength:
const collator = new Intl.Collator(['en-US'], {strength: 2});
const strings = ['résumé', 'Resume', 'resume'];
strings.sort(collator.compare);
// strings will be ['resume', 'Resume', 'résumé']
In this example, the collation strength is set to 2, which means that only primary differences (e.g. differences in base letters) are taken into account when comparing strings.
The Intl.Collator
object is useful for comparing strings in a locale-sensitive manner, and for specifying the collation strength and sensitivity when comparing strings.
Intl.DateTimeFormat
The Intl.DateTimeFormat
object that provides support for formatting and parsing dates and times according to the conventions of a specific locale.
Here’s an example of how you might use the Intl.DateTimeFormat
object to create a formatter object that formats dates and times in the en-US
locale:
const formatter = new Intl.DateTimeFormat('en-US');
const date = new Date();
const formattedDate = formatter.format(date);
// formattedDate will be a string representation of the current date and time in the 'en-US' locale
In this example, the Intl.DateTimeFormat
object is used to create a formatter object for the en-US
locale. The format
method of the formatter object is then used to format a Date
object as a string.
You can also use the Intl.DateTimeFormat
object to specify the format of the date and time string that you want to generate. For example, you can use the weekday
, year
, month
, and day
properties of the options object to specify the format of the date:
const options = {weekday: 'long', year: 'numeric', month: 'long', day: 'numeric'};
const formatter = new Intl.DateTimeFormat('en-US', options);
const date = new Date();
const formattedDate = formatter.format(date);
// formattedDate will be a string representation of the current date in the 'en-US' locale, in the format 'Wednesday, January 26, 2022'
You can also use the hour
, minute
, and second
properties of the options object to specify the format of the time:
const options = {hour: 'numeric', minute: 'numeric', second: 'numeric'};
const formatter = new Intl.DateTimeFormat('en-US', options);
const date = new Date();
const formattedDate = formatter.format(date);
// formattedDate will be a string representation of the current time in the 'en-US' locale, in the format '12:34:56'
The Intl.DateTimeFormat
object is useful for formatting and parsing dates and times in a locale-sensitive manner, and for specifying the format of the date and time string that you want to generate.
Intl.ListFormat
The Intl.ListFormat
object that provides support for formatting lists of items according to the conventions of a specific locale.
Here’s an example of how you might use the Intl.ListFormat
object to create a formatter object that formats lists of items in the en-US
locale:
const formatter = new Intl.ListFormat('de-de');
const items = ['apple', 'banana', 'orange'];
const formattedList = formatter.format(items);
// formattedList will be 'apple, banana und orange'
In this example, the Intl.ListFormat
object is used to create a formatter object for the en-US
locale. The format
method of the formatter object is then used to format an array of items as a string.
You can also use the Intl.ListFormat
object to specify the style of the list that you want to generate. For example, you can use the style
property of the options object to specify the style of the list:
const options = {style: 'long'};
const formatter = new Intl.ListFormat('de-de', options);
const items = ['apple', 'banana', 'orange'];
const formattedList = formatter.format(items);
// formattedList will be 'apple, banana und orange'
In this example, the style
property is set to long
, which means that the list will be formatted using the long form of the list separators.
You can also use the Intl.ListFormat
object to specify the type of the list that you want to generate. For example, you can use the type
property of the options object to specify the type of the list:
const options = {type: 'conjunction'};
const formatter = new Intl.ListFormat('de-de', options);
const items = ['apple', 'banana', 'orange'];
const formattedList = formatter.format(items);
// formattedList will be 'apple, banana und orange'
In this example, the type
property is set to conjunction
, which means that the list will be formatted using the conjunction form of the list separators.
The Intl.ListFormat
object is useful for formatting lists of items in a locale-sensitive manner, and for specifying the style and type of the list that you want to generate.
Intl.NumberFormat
The Intl.NumberFormat
object is a part of the Intl
object in JavaScript that provides support for formatting and parsing numbers according to the conventions of a specific locale.
Here’s an example of how you might use the Intl.NumberFormat
object to create a formatter object that formats numbers in the en-US
locale:
const formatter = new Intl.NumberFormat('en-US');
const number = 123456.789;
const formattedNumber = formatter.format(number);
// formattedNumber will be '123,456.789'
In this example, the Intl.NumberFormat
object is used to create a formatter object for the en-US
locale. The format
method of the formatter object is then used to format a number as a string.
You can also use the Intl.NumberFormat
object to specify the format of the number string that you want to generate. For example, you can use the minimumFractionDigits
and maximumFractionDigits
properties of the options object to specify the minimum and maximum number of fraction digits to include in the formatted number:
const options = {minimumFractionDigits: 2, maximumFractionDigits: 4};
const formatter = new Intl.NumberFormat('en-US', options);
const number = 123456.789;
const formattedNumber = formatter.format(number);
// formattedNumber will be '123,456.7890'
In this example, the minimumFractionDigits
and maximumFractionDigits
properties are set to 2 and 4, respectively, which means that the formatted number will include at least 2 and at most 4 fraction digits.
You can also use the Intl.NumberFormat
object to specify the style of the number that you want to generate. For example, you can use the style
property of the options object to specify the style of the number:
const options = {style: 'currency', currency: 'USD'};
const formatter = new Intl.NumberFormat('en-US', options);
const number = 123456.789;
const formattedNumber = formatter.format(number);
// formattedNumber will be '$123,456.79'
In this example, the style
property is set to currency
, which means that the number will be formatted as a currency value in the specified currency (in this case, US dollars).
The Intl.NumberFormat
object is useful for formatting and parsing numbers in a locale-sensitive manner, and for specifying the format and style of the number that you want to generate.
Intl.PluralRules
The Intl.PluralRules
object that provides support for determining the appropriate plural form to use for a number according to the conventions of a specific locale.
Here’s an example of how you might use the Intl.PluralRules
object to create a plural rules object for the en-US
locale:
const pluralRules = new Intl.PluralRules('en-US');
Once you have created a plural rules object, you can use the select
method to determine the appropriate plural form to use for a given number:
const pluralForm = pluralRules.select(1);
// pluralForm will be 'one'
const pluralForm = pluralRules.select(2);
// pluralForm will be 'other'
In this example, the select
method is used to determine the appropriate plural form to use for the numbers 1 and 2 in the en-US
locale. The select
method returns the string 'one' for the number 1, and 'other' for the number 2.
You can also use the Intl.PluralRules
object to specify the type of the number that you want to use when determining the plural form. For example, you can use the type
property of the options object to specify the type of the number:
const options = {type: 'cardinal'};
const pluralRules = new Intl.PluralRules('en-US', options);
const pluralForm = pluralRules.select(1);
// pluralForm will be 'one'
In this example, the type
property is set to cardinal
, which means that the plural rules object will use cardinal numbers when determining the plural form
Intl.RelativeTimeFormat
The Intl.RelativeTimeFormat
object is a part of the Intl
object in JavaScript that provides support for formatting relative times (e.g. "in 5 minutes") according to the conventions of a specific locale.
Here’s an example of how you might use the Intl.RelativeTimeFormat
object to create a formatter object for the en-US
locale:
const formatter = new Intl.RelativeTimeFormat('en-US');
Once you have created a formatter object, you can use the format
method to format a relative time:
const relativeTime = formatter.format(-5, 'minute');
// relativeTime will be '5 minutes ago'
const relativeTime = formatter.format(5, 'minute');
// relativeTime will be 'in 5 minutes'
In this example, the format
method is used to format the relative times -5 minutes and 5 minutes in the en-US
locale. The format
method takes a number and a unit (e.g. 'minute', 'hour', 'day') as input, and returns a string representation of the relative time.
You can also use the Intl.RelativeTimeFormat
object to specify the style of the relative time string that you want to generate. For example, you can use the style
property of the options object to specify the style of the relative time:
const options = {style: 'short'};
const formatter = new Intl.RelativeTimeFormat('en-US', options);
const relativeTime = formatter.format(-5, 'minute');
// relativeTime will be '-5 min'
In this example, the style
property is set to short
, which means that the relative time will be formatted using the short form of the relative time string.
The Intl.RelativeTimeFormat
object is useful for formatting relative times in a locale-sensitive manner, and for specifying the style of the relative time string that you want to generate.
Intl.Segmenter
The Intl.Segmenter
object in JavaScript that provides support for segmenting text into logical units (e.g. words, sentences, or paragraphs) according to the conventions of a specific locale.
Here’s an example of how you might use the Intl.Segmenter
object to create a segmenter object for the ja-JP
locale:
const segmenter = new Intl.Segmenter('ja-JP');
In this example, the segmenter object is created for the ja-JP
locale, which is the Japanese language as used in Japan.
You can then use the segment
method to segment a string of text into logical units, just as in the previous example:
const iterator = segmenter.segment('これは文です。これは別の文です。');
for (const unit of iterator) {
console.log(unit);
}
In this example, the segment
method is used to segment a string of text (written in Japanese) into sentences. The segment
method returns an iterator that you can use to iterate over the segments of the text.
The Intl.Segmenter
object can be used to segment text into logical units in any locale, not just en-US
and ja-JP
. You can specify any valid locale when creating a segmenter object, and the segment
method will use the conventions of that locale to segment the text.
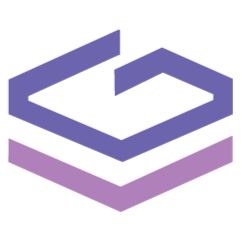
Write a comment ...